AppsFlyer
Learn how to set up integration with AppsFlyer
AppsFlyer is a leading platform for mobile attribution and marketing analytics. It stands as a third-party service that gathers and organizes data from marketing campaigns. This helps companies see how well their campaigns are performing in one place.
Adapty provides a complete set of data that lets you track subscription events from stores in one place. With Adapty, you can easily see how your subscribers are behaving, learn what they like, and use that information to communicate with them in a way that's targeted and effective. Therefore, this integration allows you to track subscription events in AppsFlyer and analyze precisely how much revenue your campaigns generate.
The integration between Adapty and AppsFlyer operates in two main ways.
- Receiving attribution data from AppsFlyer
Once you've set up the AppsFlyer integration, Adapty will start receiving attribution data from AppsFlyer. You can easily access and view this data on the user's profile page.
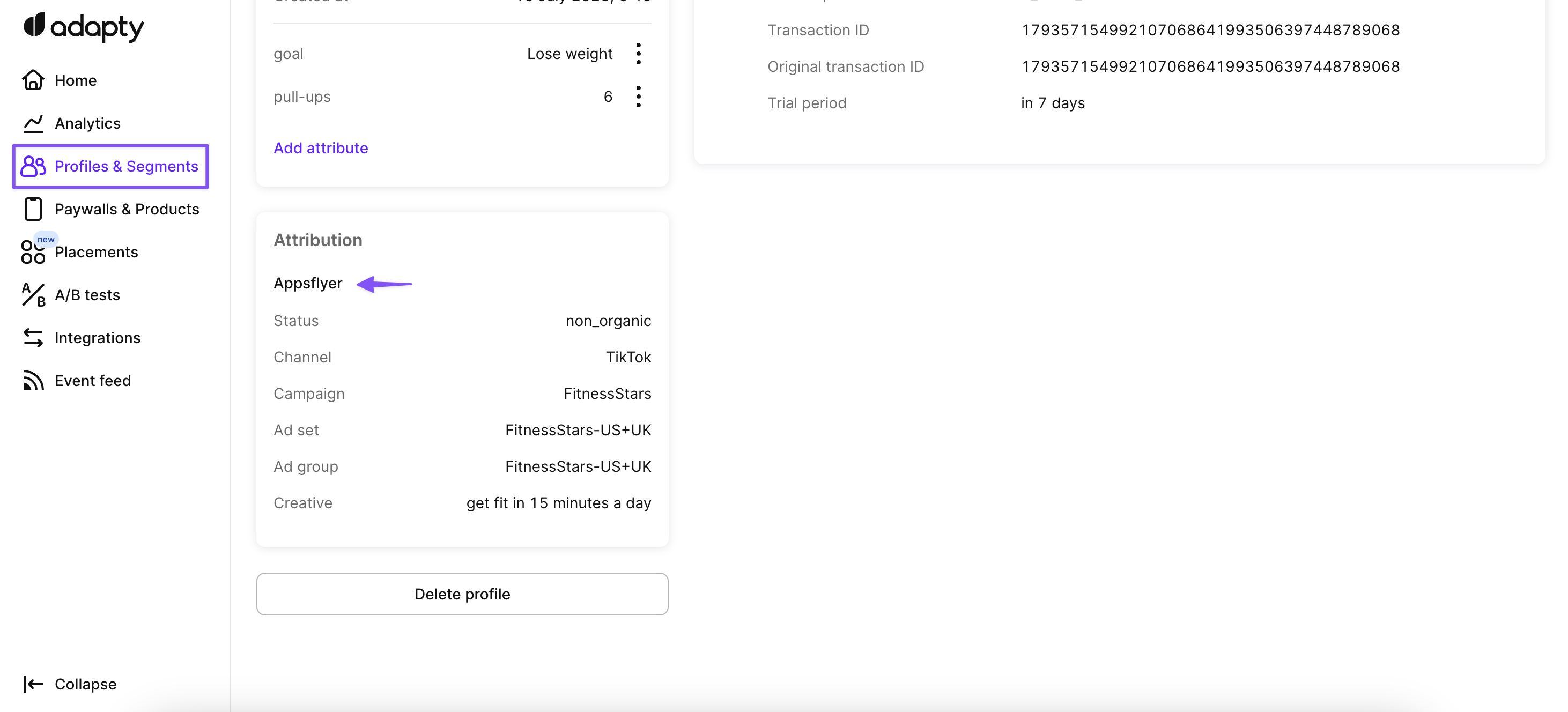
- Sending subscription events to AppsFlyer
Adapty can send all subscription events which are configured in your integration to AppsFlyer. As a result, you'll be able to track these events within the AppsFlyer dashboard. This integration is beneficial for evaluating the effectiveness of your advertising campaigns.
How to set up AppsFlyer integration
To setup the integration with AppsFlyer go to Integrations > AppsFlyer in the Adapty Dashboard, turn on a toggle from off to on, and fill out fields.
The next step of the integration is to set credentials.
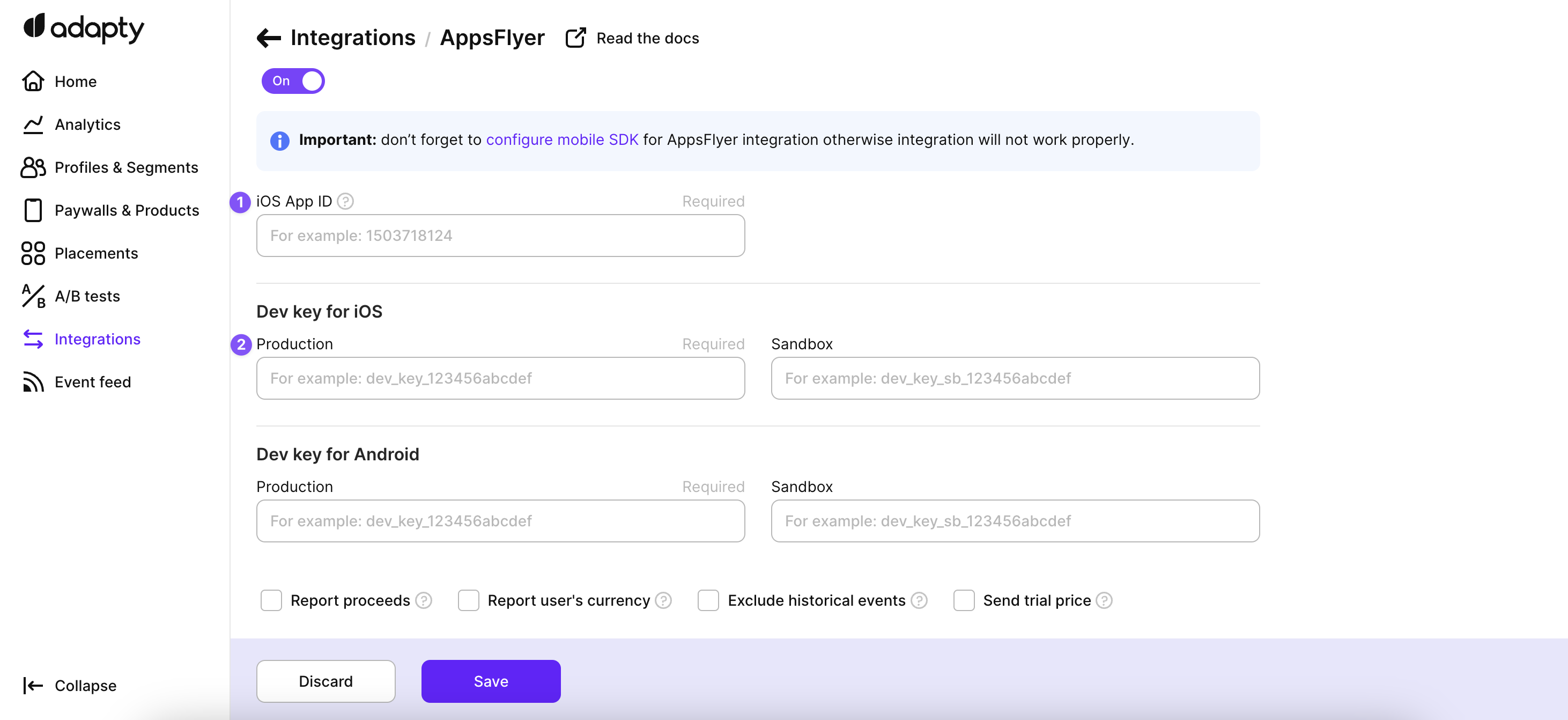
- To find App ID, open your app page in App Store Connect, go to the App Information page in section General, and find Apple ID in the left bottom part of the screen.
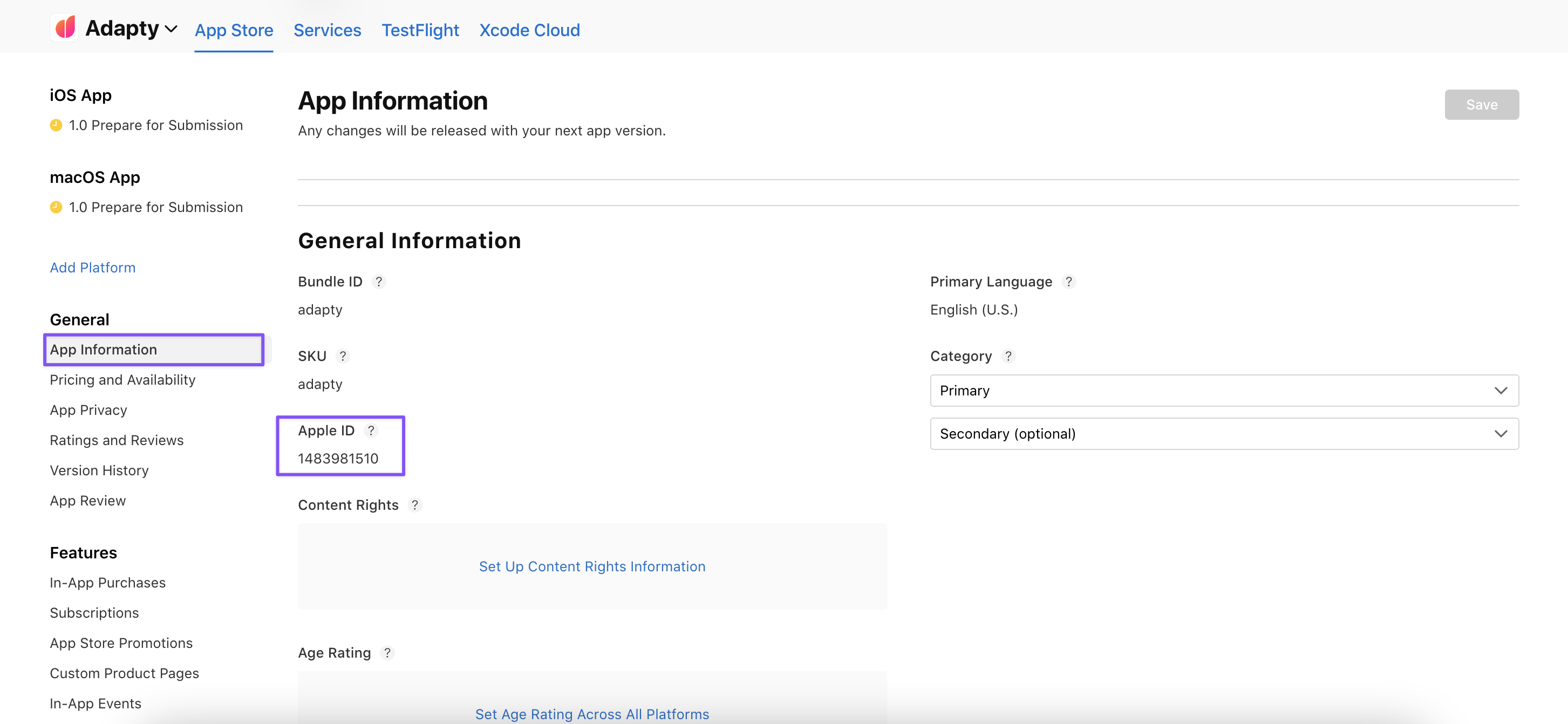
- Open AppsFlyer and navigate to your app page. Scroll the left menu bar, find App Settings, and copy the Dev Key. Then you need to use the value Dev Key for your iOS and Android apps in the Adapty Dashboard.
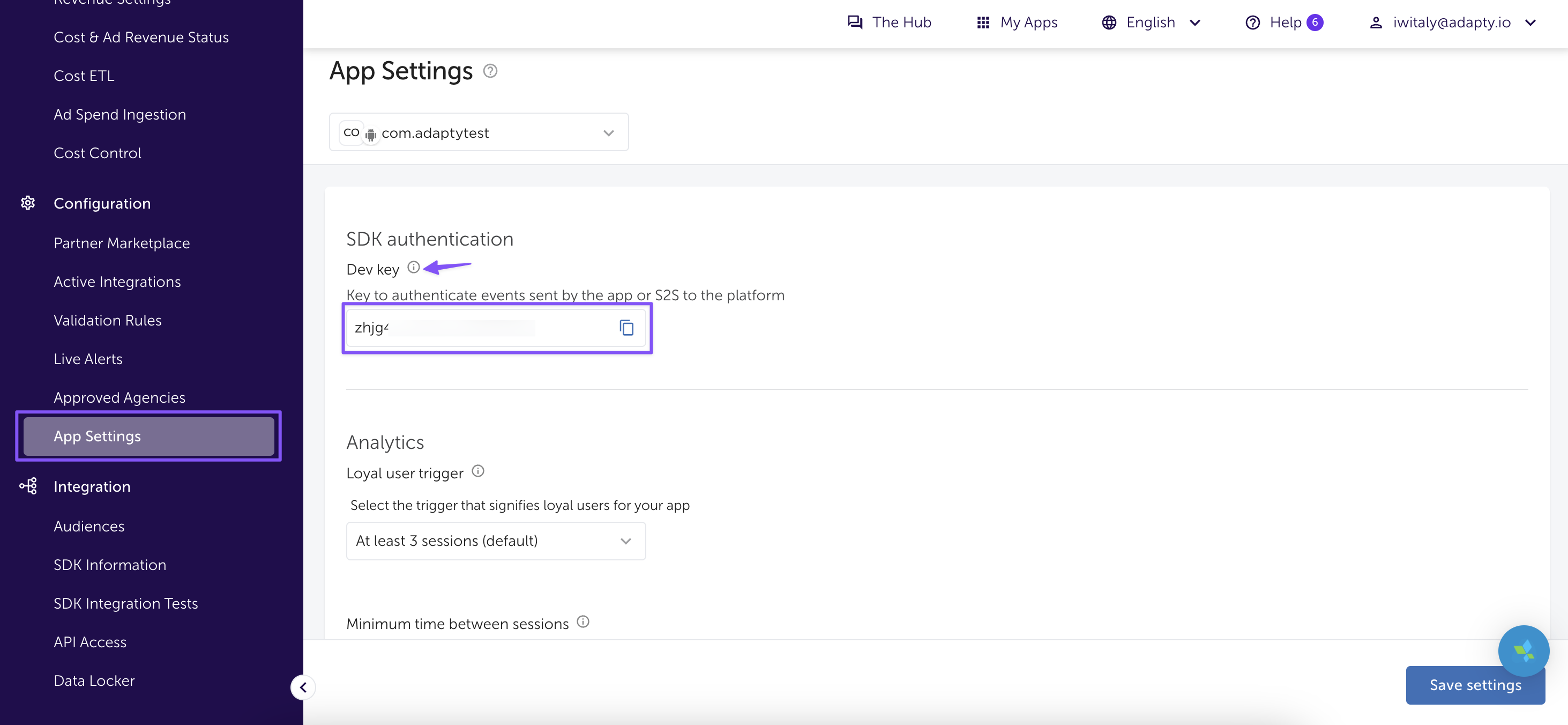
App Settings page on AppsFlyer dashboard
AppsFlyer doesn't have a Sandbox mode for server2server integration. So you need a different application/account in AppsFlyer for Sandbox Dev Key. If you want to send sandbox events to the same app, just use the same key for production and sandbox.
Adapty maps some events to AppsFlyer standard events by default. With such configuration, AppsFlyer can further send events to each ad network that you use without additional setup.
Another important thing is that AppsFlyer doesn't support events older than 24 hours. So, if you have an event that is more than a day old, Adapty will send it to Appsflyer, but the event datetime will be replaced by the current timestamp.
Events and tags
Below the credentials, there are three groups of events you can send to AppsFlyer from Adapty. Simply turn on the ones you need. Check the full list of the events offered by Adapty here.
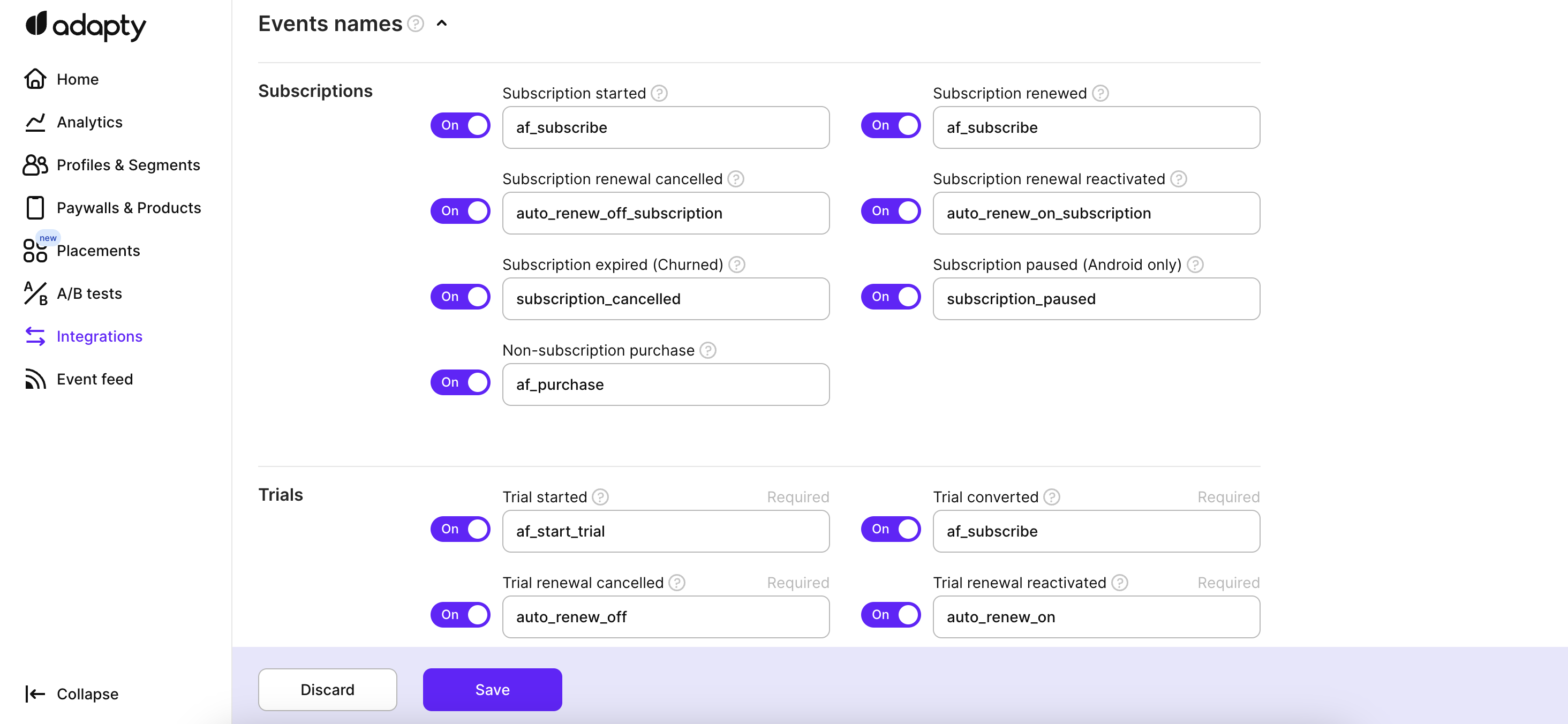
Subscriptions, trials, issues events in Adapty integration interface
We recommend using the default event names provided by Adapty. But you can change the event names based on your needs.
Adapty will send subscription events to AppsFlyer using a server-to-server integration, allowing you to view all subscription events in your AppsFlyer dashboard and link them to your acquisition campaigns.
SDK configuration
It's very important to send AppsFlyer attribution data from the device to Adapty using Adapty.updateAttribution()
SDK method. The example below shows how to do that.
// Find your implementation of AppsFlyerLibDelegate
// and update onConversionDataSuccess method:
func onConversionDataSuccess(_ installData: [AnyHashable : Any]) {
// It's important to include the network user ID
Adapty.updateAttribution(installData, source: .appsflyer, networkUserId: AppsFlyerLib.shared().getAppsFlyerUID())
}
val conversionListener: AppsFlyerConversionListener = object : AppsFlyerConversionListener {
override fun onConversionDataSuccess(conversionData: Map<String, Any>) {
// It's important to include the network user ID
Adapty.updateAttribution(
conversionData,
AdaptyAttributionSource.APPSFLYER,
AppsFlyerLib.getInstance().getAppsFlyerUID(context)
) { error ->
if (error != null) {
//handle error
}
}
}
}
import 'package:appsflyer_sdk/appsflyer_sdk.dart';
AppsflyerSdk appsflyerSdk = AppsflyerSdk(<YOUR_OPTIONS>);
appsflyerSdk.onInstallConversionData((data) async {
try {
// It's important to include the network user ID
final appsFlyerUID = await appsFlyerSdk.getAppsFlyerUID();
await Adapty().updateAttribution(
data,
source: AdaptyAttributionSource.appsflyer,
networkUserId: appsFlyerUID,
);
} on AdaptyError catch (adaptyError) {
// handle error
} catch (e) {}
});
appsflyerSdk.initSdk(
registerConversionDataCallback: true,
registerOnAppOpenAttributionCallback: true,
registerOnDeepLinkingCallback: true,
);
import { adapty, AttributionSource } from 'react-native-adapty';
import appsFlyer from 'react-native-appsflyer';
appsFlyer.onInstallConversionData(installData => {
try {
// It's important to include the network user ID
const networkUserId = appsFlyer.getAppsFlyerUID();
adapty.updateAttribution(installData, AttributionSource.AppsFlyer, networkUserId);
} catch (error) {
// handle error
}
});
// ...
appsFlyer.initSdk(/*...*/);
using AppsFlyerSDK;
// before SDK initialization
AppsFlyer.getConversionData(this.name);
// in your IAppsFlyerConversionData
void onConversionDataSuccess(string conversionData) {
// It's important to include the network user ID
string appsFlyerId = AppsFlyer.getAppsFlyerId();
Adapty.UpdateAttribution(conversionData, AttributionSource.Appsflyer, appsFlyerId, (error) => {
// handle the error
});
}
Updated 10 days ago